Table of Contents
Selenium Webdriver Script in Java
Following is the sample selenium webdriver script written in Java that uses most common selenium webdriver commands. It will load the URL “https://siteforinfotech.com” on the chrome browser, navigates to “Contact Us” page, fills the fields of the forms and submit it, then refreshes the page and go back to the previous page.
You can copy the code and paste it to run on the Java class that you have created with the help of the previous post written
package selenium_package;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class Selenium_Class {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
// launch Google Chrome and direct it to the provided URL
driver.get("https://siteforinfotech.com/");
// verify the title of the page
if(driver.getTitle().contentEquals("InfoTechSite | CS/IT Tutorials, MCQs, Guides And Reviews")) {
System.out.println("Title is correct");
}
else {
System.out.println("Title is incorrect");
}
// finds the link "contact us" and click on it
driver.findElement(By.linkText("Contact Us")).click();
// gets the title of the page and print it
System.out.println(driver.getTitle());
// uses findElement By Name and uses sendKeys to fill the form
driver.findElement(By.name("your-name")).sendKeys("Your Name");
driver.findElement(By.name("your-email")).sendKeys("[email protected]");
driver.findElement(By.name("your-subject")).sendKeys("Write you subject here");
// uses findElement By cssSelector and clicks on submit button
driver.findElement(By.cssSelector("input[type=submit]")).click();
// WebDriver pauses for 10 seconds
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// uses navigate commands to refresh and go back
driver.navigate().refresh();
driver.navigate().back();
System.out.println("Test Passed!");
// closes the test window
driver.close();
}
}
Following is the quick introduction of the selenium webdriver scripts used on the Java class written above.
The first block of the codes started with import keyword will import required packages to the class. These packages will instantiate a new browser which will load required drivers.
The line of code “WebDriver driver = new ChromeDriver();” instantiates driver objects for Google chrome driver. When there is no parameters are added the browser will be launched on default mode.
The “driver.get()” command used just below the driver object opens the provided URL on the google chrome browser. The “driver.getTitle()” command retrieves the actual title of the page and “contentEquals()” method will compare with the expected title in order to verify the title of the page.
I have written “driver.findElement(By.linkText(“Contact Us”)).click();” command to find out the desired link and execute the click() command on it. “findElement(By.name())” method wil find the desired elements by name and “sendkeys()” fills the input field.
The command “driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);” holds the testing process for 10 seconds. “navigate().refresh()” and “navigate().back()” are the browser navigation commands that refreshes and return back to the previous page. The last command “driver.close();” closes the browser opened by the webdriver.
Refer to our next post about the selenium webdriver commands to get more information about most commonly used selenium webdriver commands.
Running The Script for Firefox
In order to run the selenium webdriver script for Firefox browser, you have to add or replace the following codes.
Import the Firefox driver on the import block for automating the webpage on Firefox browser. Use the following line of code for importing the Firefox driver.
import org.openqa.selenium.firefox.FirefoxDriver;
For the latest version of Firefox, you need to use gecko driver created by Mozilla. It should be used before instantiating driver object. For this down the gecko driver from GitHub and extract the compressed “.zip” file on any location of your drive i.e. “E:\geckdriver.exe“. Now use this driver file with “System.setproperty()” method as given below.
System.setProperty("webdriver.firefox.gecko.driver","E:\\geckodriver.exe");
WebDriver driver = new FirefoxDriver();
Running the Script for IE
Same as running the selenium webdriver script for Firefox browser, you can run the script for IE also with making little change on the following lines of codes from the Java class given above.
At first, import the Internet Explorer driver server on the import block for automating the webpage on IE browser. Use the following line of code for importing the Internet Explorer driver server.
import org.openqa.selenium.ie.InternetExplorerDriver;
Now, download the Internet Explorer driver server file from the selenium website as shown on the image below. The driver server files are available for both 32-bit windows and 64-bit windows.
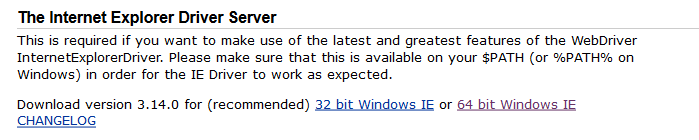
Now extract the compressed “.zip” file on any location of your drive i.e. “E:\IEDriverServer.exe” and use the extracted driver file with “System.setProperty()” method as given below.
System.setProperty("webdriver.ie.driver","E:\\IEDriverServer.exe");
WebDriver driver = new InternetExplorerDriver();
When there was an error while running the selenium webdriver script on the IE browser, verify the following settings on internet options security.
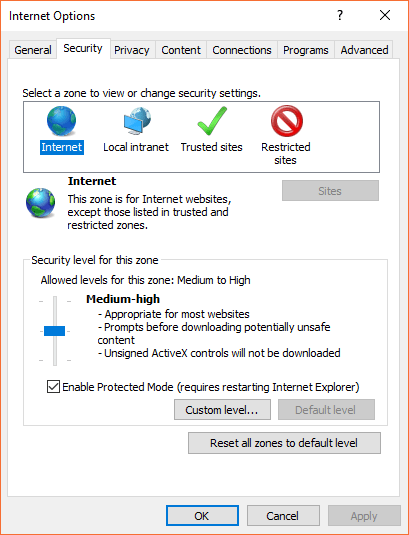
For this, go to IE toolbar, click
Read Next: Selenium Webdriver Commands Required for Automated Testing
Comments are closed.